How to Get the Length of a List in Python
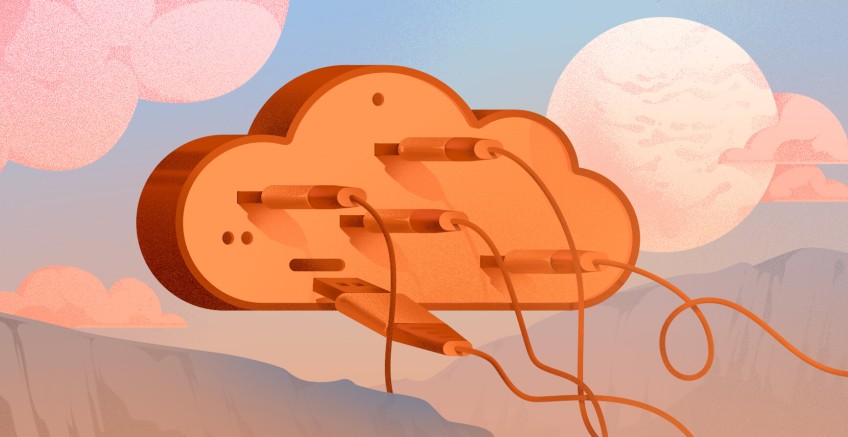
You can use multiple techniques to find the length of a list in Python. In this article, you will learn what lists in Python are, how to get the length of a list in Python using different methods, and why you should use them. We describe five different ways with examples.
#What are lists in Python?
Python lists are a collection of ordered and mutable items, represented by square brackets []
. Getting the length of a list in Python is a standard operation you‘ll often encounter when working with lists. The length of a list is simply the number of elements or items it contains.
#How to use lists in Python?
Python lists are very important in solving coding problems for being iterable and for their capability to carry different data types. Lists are very flexible and support many common operations in Python, including checking the length of a list, appending to a list, inserting into a list, sorting, reversing, combining two lists, or indexing and accessing elements.
Below, we will focus on how to get the length of a list in Python. Before delving into how to find the length of a list in Python, let’s go over the prerequisites.
#Prerequisites
Note: Manage your cloud servers and network directly from Python code with Cherry Servers Python API integration. Learn more about our API and integrations here.
Deploy and scale your Python projects effortlessly on Cherry Servers' robust and cost-effective dedicated or virtual servers. Benefit from an open cloud ecosystem with seamless API & integrations and a Python library.
#How to get the length of a list in Python: 5 methods
We will focus on five different methods that can be used to find the length of a list in Python. Here are some of the most common methods in Python to check the length of a list:
- The
len()
methods. - Using for loop method (naive method).
- Using the
length_hint()
function. - Using
__len__()
special function. - Using the NumPy library.
Let's review each of them in detail.
#1. The len() function: What is len in Python?
The most common way to get the Python list length is through the built-in function len()
. Len in Python is a function that takes in a single argument and returns the number of elements or items in that argument. The argument can be any iterable: either a dictionary, a tuple, a list or even a custom object. In this article we will be focusing on the list.
The code syntaxt are as follows:
len(name_of_list)
In the code below, a list with a few integers named quarterly_revenue
was created. It is then passed as an argument to the len()
function to get its length. The output is then stored in the variable named length_list
which is eventually printed out to the terminal.
#Initial creation of the list containing different integers
quarterly_revenue = [15000,12511,12446,245424,286,248]
#Storing the result in a variable
length_list = len(quarterly_revenue)
#Printing out the variable
print(length_list)
#After running the code the result:
#6
In another example below, a list list_of_things
was created containing different data types such as a string, an integer, a Boolean and a float. The list is passed as an argument to the len()
function, and the result is stored in a variable length_list
. Eventually, the output is printed out to the terminal.
#Initial creation of the list containing strings, integers, boolean and float
list_of_things = [1500, "sodas", True, 1.5]
#Storing the result in a variable
length_list = len(list_of_things)
#Printing out the variable
print(length_list)
#After running the code the result:
#4
#2. Using the for
loop in Python
Another way to get the length of a list in Python is to use a for loop to iterate over the elements of the list and count them. This method is also known as a naive method.
Using the Python for loop method is not as straightforward and common as the len()
function. Take a look at the example below.
A variable count
is set to a value of 0
and the for
loop iterates over the list quarterly_revenue
array. The value of the variable is then incremented by 1 for each element until the loop stops and the output is stored in the count
variable. Its value is then printed out to the terminal.
Quarterly_revenue = [15000,12511,12446,245424,286,248]
#Initialising a variable and set its value to 0
count = 0
#calling a for loop and iterating over each integer in the list
for number in Quarterly_revenue:
#then adding +1 over each value iterated over.
count += 1
#Setting a print function to print out the updated variable count
print(count)
#3. Using the length_hint()
function in Python
You can also find the length of a list by using the length_hint()
function. It is used to get an estimated length of a large iterable like a generator or a custom object. It is primarily used to estimate the amount of memory that needs to be pre-allocated for large iterables. Nevertheless, you may use length_hint()
to find the length of a list as well, since it first calls the __len__
method under the hood, which is implemented by default for a Python list.
The syntax for the code can be seen below.
from operator import length_hint
length_hint(name_of_list)
In the example below the length_hint()
function was imported from a built-in operator
module. The list quarterly_revenue
was passed as an argument to the length_hint()
and the output was then stored in a variable length_list
. The output was then printed out to the terminal.
#To use the length_hint function it has to be imported from the operator module.
from operator import length_hint
#The list we intend to find its length
quarterly_revenue = [15000,12511,12446,245424,286,248]
#Passing the list created into the length_hint() function as an argument and the ouput stored in a variable
length_list = length_hint(quarterly_revenue)
print(length_list)
#Output: 6
#4. Using a __len__()
special function
A __len__
function is a special method in Python that is used to implement the inbuilt len()
function.
Under the hood when running the len()
function to determine the length of an object, it checks for the __len__
special method in that object. If the __len__
special method is present, len()
calls it and returns the length of that object. Otherwise, a typerror
is raised.
The syntax for the code can be seen below:
name_of_list.__len__()
In the code below, the special method __len__()
was called on the list quarterly_revenue
containing different integers created and the output was stored in a variable and the result was printed out.
#list containing integers
quarterly_revenue = [15000,12511,12446,245424,286,248]
# getting list length using the __len__() method and storing it inside a variable
list_length = quarterly_revenue.__len__()
# Printing out the length of the list
print(list_length)
#5. NumPy in Python: Using a NumPy library
Python lists are very versatile, but not as fast as the ndarray
data type of a NumPy library. The goal of NumPy is to offer array objects that are up to 50 times faster than conventional Python lists.
The NumPy array object is referred to as ndarray
, and it has a number of supporting methods that make using ndarray
relatively simple. In data research, where speed and resources are crucial, ndarray
data type is employed a lot.
NumPy also provides a function called size()
that can be used to determine the length of an array or a list.
To use NumPy you have to install the NumPy package using pip(pip install packages) available from Python.
pip install numpy
In the example below the NumPy library was imported after the installation has been completed, the list quarterly_revenue
was passed into the size()
function and the output was stored in the variable length_list
which is then printed out.
#importing numpy as np you can import it as anything but this is just the conventional way
import numpy as np
#The list containing different range of integers
quarterly_revenue = [15000,12511,12446,245424,286,248]
# getting list length using the __len__() method and storing it inside a variable
length_list = np.size(quarterly_revenue)
print(length_list)
Discover how Caxita, an online travel engine application company with high workloads, eliminated downtime and allowed engineers to focus on development thanks to Cherry Servers' robust bare metal cloud and 24/7 technical support.
#What is the best way to get the length of a list in Python?
There are various reasons why you should choose one length method over another. One of the main criteria is often performance.
In this section, you will take a look at which of the different methods executes faster. Being able to estimate the code's execution time is a smart programming skill. This will help you make a proper choice about which method you should use when solving a specific problem.
The code below shows the analysis of the amount of time it will take to find the length of a list using different length methods. The imported time
library will be used to determine the start
time before the function starts and the end
time after the code finishes running. The list quarterly_revenue
was passed into the various methods with each start
and end
time recorded consecutively.
# Import time, length_hint and numpy modules
import time
from operator import length_hint
import numpy as np
# Create a list containing integers
Quarterly_revenue = [15000,12511,12446,245424,286,248]
#Print out the list items
print('The items in the list are: ' + str(quarterly_revenue))
# 1. Using a built-in len() function
# Take note of the start time
begin_time_len = time.perf_counter()
# Use the built-in len() function
length_of_list = len(quarterly_revenue)
# Take note of the end time to determine execution time
end_time_len = str(time.perf_counter() - begin_time_len)
# 2. Using a for loop
# Take note of the start time
begin_time_constraint = time.perf_counter()
# Initialize length as 0 and use the for loop
length = 0
for _ in quarterly_revenue:
length = length + 1
# Take note of the end time to determine execution time
end_time_constraint = str(time.perf_counter() - begin_time_constraint)
# 3. Using a length_hint() method
# Take note of the start time
begin_time_hint = time.perf_counter()
# Use the length_hint() method
length_hint_list = length_hint(quarterly_revenue)
# Take note of the end time to determine execution time
end_time_hint = str(time.perf_counter() - begin_time_hint)
# 4. Using a NumPy module
# Take note of the start time
begin_time_numpy = time.perf_counter()
# Use the NumPy module's size() method
length_numpy_list = np.size(Quarterly_revenue)
# Take note of the end time to determine execution time
end_time_numpy = str(time.perf_counter() - begin_time_numpy)
# Print out all the results
print('len() method execution time: ' + end_time_len)
print('for loop execution time: ' + end_time_constraint)
print('length_hint() method execution time: ' + end_time_hint)
print('NumPy module execution time: ' + end_time_numpy)
The image below indicates the time taken for the various methods where length_hint()>>naive >>numpy>>len(). As you can see, the len()
method took the least time to find the length of the list. It is important to note that the execution time may vary based on several different parameters like the operating system load. It means that in two consecutive runs you may get contrasting results.
#Conclusion
This tutorial has walked you through five different methods on how to find the length of a list in Python: len()
, for loop, length_hint()
, __len__()
special function and the NumPy library. Knowing many techniques gives you the freedom to select the most appropriate one for your use case.
At this point you should be confident enough to implement what you have learned in solving your own coding problems.
Cloud VPS Hosting
Starting at just $3.24 / month, get virtual servers with top-tier performance.