How to Add Elements to a List in Python? | 4 Methods
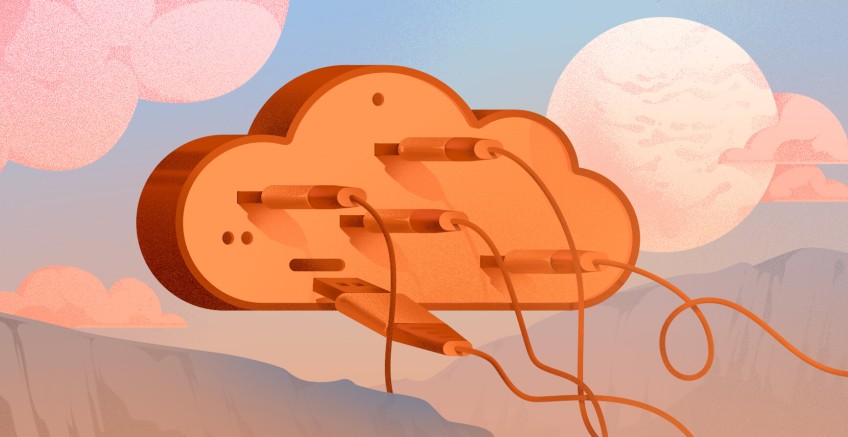
Python lists are used to store an ordered collection of items. They can hold values of the same data type or heterogeneous values, such as strings and integers within the same list. This detailed tutorial shows you four different ways to add elements to a list in Python.
#What are Lists in Python?
In Python, a list is a collection of comma-separated values or items enclosed inside square brackets [ ]. It stores values of the same or different data types, as earlier mentioned. For example, you can have a list of strings only or a mix of both integers and strings.
Below is a Python list example that stores string values.
pets = ['Cat', 'Dog', 'Parrot', 'Hamster']
Elements inside a list can be accessed using integer indices where the first element takes the index of 0, and the second element takes the index of 1, and so on. This stems from the fact that lists are ordered, and elements retain the order in which they appear.
Python provides various ways of working and manipulating lists. You can perform various operations, such as adding, removing, or changing elements inside a list.
Let’s explore various ways of adding elements to a list in Python.
#How to add elements to a list in Python?
Data structures are one of the core concepts of programming. They provide a means of organizing data for efficient storage and access.
Python provides four main data structures, which are broadly categorized into mutable (lists, sets, and dictionaries) and immutable (tuples) data types. Mutable data types can be modified or changed, while immutable ones are static and cannot be altered.
There are four ways of adding elements or values to an existing list in Python. Let’s check them out.
#Prerequisites
To follow along in this guide, you need to have Python installed on your machine. In this tutorial, we are working with Python 3.11. In addition to installing Python, it is recommended to have an IDE such as Pycharm installed to make writing code easier and more efficient.
Deploy and scale your Python projects effortlessly on Cherry Servers' robust and cost-effective dedicated or virtual servers. Benefit from an open cloud ecosystem with seamless API & integrations and a Python library.
#Method 1: Add items to a list using the append() method
The append()
method is used to add values at the end of a list. It adds a new element at the end of a list, right after its last item. Consider a list called cars
that contains a collection of car models as shown:
cars = ["Nissan", "Ford", "Mercedes", "Mazda"]
To add another car model to the list, say Toyota
, call the append()
method on the list with Toyota
as the parameter.
cars.append(“Toyota”)
Now display the list of items using the print function:
print(cars)
Output
['Nissan', 'Ford', 'Mercedes', 'Mazda', 'Toyota']
From the output, you can see that Toyota
has been placed at the end of the list.
#Method 2: Add items at a specific index in a list
The insert()
method lets you add an item at a specific index in the list. As you know, list elements are ordered, starting with index 0 for the first item.
The insert()
method takes two parameters: the index of the new element to be added and the value of the element.
Using the cars
list, let us now insert Toyota
at index 1. To do so, run the following code:
cars.insert(1, “Toyota”)
Display the list items as before.
print(cars)
Output
['Nissan', 'Toyota', 'Ford', 'Mercedes', 'Mazda']
This time around, the new item Toyota
appears at index 1, just like we had specified in the insert()
method.
#Method 3: Extend a list from an iterable using the extend() method
The extend()
method appends list elements from an iterable such as another list, tuple, or set to the end of the existing list. Suppose you have two lists that store a collection of fruits, as shown.
myFruits_list1 = ["Apples", "Oranges", "Mangoes"]
myFruits_list2 = ["Strawberries", "Bananas", "grapes"]
To add the elements of myFruits_list1
to myFruits_list2
call the extend()
method as follows.
myFruits_list2.extend(myFruits_list1)
This adds all the items in the first list to the end of the second list.
print(myFruits_list1)
Output
['Strawberries', 'Bananas', 'grapes', 'Apples", 'Oranges', 'Mangoes']
As we mentioned earlier, you can extend a list using elements contained in a tuple. Take the following example.
Here, we have a list called furniture
and a tuple called points
.
furniture = ['Sofas', 'beds', 'tables', 'chairs']
points = (1, 4, 5, 9)
To add the values in the points
tuple to the furniture
list, call the extend()
method as follows.
furniture.extend(points)
To verify this, run the print()
function as shown.
print(furniture)
The furniture
list, now contains additional elements, which are integer values from the points
tuple.
Output
['Sofas', 'beds', 'tables', 'chairs', 1, 4, 5, 9]
Discover how Caxita, an online travel engine application company with high workloads, eliminated downtime and allowed engineers to focus on development thanks to Cherry Servers' robust bare metal cloud and 24/7 technical support.
#Method 4: List concatenation
Lastly, you can add elements to a list by concatenating two lists using the plus [ + ] operator.
Consider the following two lists:
prime_numbers = [1, 3, 5, 7, 11]
even_numbers = [2, 4, 6, 8, 10]
You can concatenate the two lists using the plus sign operator and save the result in a new list. The newly created list will comprise all elements from both lists.
new_list = prime_numbers + even_numbers
``
Then view the new list.
```python
print(new_list)
Output
[1, 3, 5, 7, 11, 2, 4, 6, 8, 10]
#Conclusion
In this tutorial, you have learned different ways of adding elements to a list in Python. As you’ve seen, different approaches will yield different outcomes. The insert()
is more specific on where exactly you want to add the new element to the list. The append()
and extend()
methods will place values at the end of the list, as does list concatenation.
Cloud VPS Hosting
Starting at just $3.24 / month, get virtual servers with top-tier performance.