How to Convert String to int in Python | Comprehensive Guide
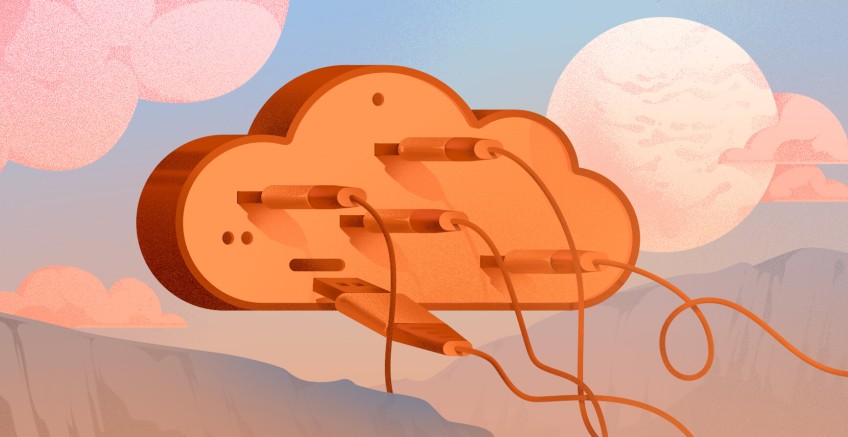
When writing code, we often need to convert values from one data type to another. For example, sometimes you receive numbers in text format. In that case, you have to convert them into integer data types to perform any mathematical operations.
Every programming language provides options to convert a string into an integer data type. So does Python. This guide explores how to convert string to int in Python, exploring these methods and discussing their key differences in detail.
#What is a string in Python?
Strings or texts in Python are stored as an ‘str’ data type. They are sequences of characters enclosed in single quotes ('') or double quotes (""). They are immutable, meaning that once created, their contents cannot be modified.
Here is how you represent strings in Python.
text1 = 'Hello Python'
text2 = "Python strings"
print(text)
print(message)
#What is int in Python?
Integers or int
data types represent whole numbers without fractional parts such as -5, +5, and 0. Integers are used for mathematical operations such as addition, subtraction, multiplication, division, and comparison.
Here is how you represent integers in Python.
integer = 100
print(integer)
print(type(integer))
Deploy and scale your Python projects effortlessly on Cherry Servers' robust and cost-effective dedicated or virtual servers. Benefit from an open cloud ecosystem with seamless API & integrations and a Python library.
#When do you need to convert string to int in Python?
When you work as a programmer, there are many occasions when you need to convert strings into an int
data type. These are some common instances:
- When you have to process user inputs, Converting user-entered string values to integers is common when handling numerical input for calculations or validations.
- When reading data from files, converting string representations of numbers to integers allows for numerical operations and analysis.
- Converting strings to integers enables numerical comparisons and sorting operations based on their numeric values rather than lexicographical order.
- Converting string data to integers is often necessary for proper indexing, filtering, and mathematical operations on numerical fields when working with databases.
#Three main ways to convert string to int in Python
int()
constructoreval()
functionast.literal_eval()
function
#1. Using Python int()
constructor
This is the most common method for converting strings into integers in Python. It's a constructor of the built-in int class rather than a function. When you call the int()
constructor, a new int
object is created.
It has the following syntax. As you can see, the default base is 10.
Syntax - int(y, base=10)
We can easily pass a string value and convert it to an int
value in this way.
number = int('10')
print("string to positive integer- ", number)
number = int("-10")
print("string with negative integer - ", number)
You can use this method even to convert float numbers into an int
data type.
number = int(10.99)
print("float to int - ",number)
However, if you pass a non-decimal integer without specifying a base, you will get an error.
int("2A3")
As we already told earlier, the default base for the int()
constructor is decimal. Therefore, int()
does not know how to parse the string. Now let us see how to mention the correct base and get the intended decimal integer as the output.
hexValue = "2A3"
print("intended Hexa Decimal result of 2A3 is - ",int(hexValue, base=16))
Also, a logical error can occur when you pass a string representing a number in a different base to int()
, but you fail to specify the appropriate base. In this case, int()
will convert the string to decimal without syntax errors, even though you intended a different base. As a result, you will receive a base 10 result instead of the expected base due to the missing base specification.
Below is an example of a logical error.
binary = "1000110100"
print("the intended result is 564 - ",int(binary))
hexadecimal = "234"
print("the intended result is 564 - ",int(hexadecimal))
Therefore, it's crucial to mention the base when using the int() constructor.
binary = "1000110100"
print("intended decimal result of bi-1000110100 is 564 - ",int(binary, base=2))
octal = "1064"
print("intended decimal result of oct-1064 is 564 - ",int(octal, base=8))
hexadecimal = "234"
print("intended decimal result of hex-234 is 564 - ",int(hexadecimal, base=16))
One of the most common exceptions that come with string-to-integer conversions is ValueError.
int()
is very limited when it comes to expressions it can parse. If it receives an input string that cannot be converted to an integer due to an incompatible form, it will raise a ValueError
exception. Therefore, it's recommended to use a validation method or try-catch
block when using int()
for user-entered strings.
#Using isdigit() for validation
You can use isdigit()
to check if a string consists only of digits, which can be useful for validating if the input can be converted to an integer using int()
. Here is what your code looks like.
user_input = input("Enter a number: ")
if user_input.isdigit():
number = int(user_input)
print("Converted integer:", number)
else:
print("Invalid input. Please enter a valid number.")
#Using try-except block to handle exceptions
Another option is to use a try-except block to handle exceptions that can occur due to invalid string inputs. Here is an example.
user_input = input("Enter a number: ")
try:
number = int(user_input)
print("Converted integer:", number)
except ValueError:
print("Invalid input. Please enter a valid number.")
#2. Using eval()
function
You can use the built-in eval()
to evaluate arbitrary Python expressions from string-based or compiled-code-based input. This is a good option if you need to dynamically evaluate Python expressions. When you pass a string argument to eval()
, it compiles it into bytecode and evaluates it as a Python expression. Essentially, the eval()
function allows you to convert strings to integers. Let's look at some examples.
print("10 - ",eval("10"))
print("2 x 3 - ",eval("2 * 3"))
print("2 ^ 3 - ",eval("2 ** 3"))
print("10 + 10 - ",eval("10 + 10"))
print("1 + 10 + 9 - ",eval("sum([1, 10, 9])"))
num = 1000
print("(1000 + 2) x 2 - ",eval("(num + 2) * 2"))
Just like int()
, you can use eval()
for the non-decimal string to int conversions as well. Here is an example.
hex_string = "0xFF"
oct_string = "0o77"
binary_string = "0b101010"
hex_value = eval(hex_string)
oct_value = eval(oct_string)
binary_value = eval(binary_string)
print(hex_value)
print(oct_value)
print(binary_value)
print(type(hex_value))
print(type(oct_value))
print(type(binary_value))
You can use the eval()
function to evaluate expressions that involve variables which is something you can’t do with int()
. Look at the following example.
x = 10
y = eval("x + 5")
print(y)
print(type(y))
The main problem with the eval()
function is as it executes arbitrary codes, this can bring unintended results. Among the three options we provide eval function is the least secure one. However, its power is bigger than the other two methods. Therefore, the best thing to do is to use the eval()
function with thorough input validation to ensure that user-provided input adheres to expected formats and constraints. This includes checking data types, restricting input length, and validating against specific patterns or ranges.
#3. Using ast.literal_eval
literal_eval()
is a Python function defined in the ast class, which stands for Abstract Syntax Tree. This module assists Python applications in processing trees of the Python abstract syntax grammar. It safely evaluates expression nodes or strings, which should only consist of strings, bytes, numbers, tuples, lists, dictionaries, sets, booleans, and None. Although this is not a recommended method for converting strings to integers, the function is capable of performing the conversion.
To use the function, we first need to import it. Let's take a look at the example below.
from ast import literal_eval
int = literal_eval("1100")
print(int)
print(type(int))
With this method, you can also convert other valid Python literals, such as booleans, lists, dictionaries, tuples, and more. For example, look at the example below.
from ast import literal_eval
string = "[1, 2, 3]"
list_data = literal_eval(string)
print(list_data)
However, unlike eval()
, literal_eval()
does not have the capability to evaluate mathematical expressions with operators (eg: - "7+8").
It will throw a ValueError exception.
from ast import literal_eval
string_num = "7 + 8"
integer = literal_eval(string_num)
print(integer)
You will need to use try-except
blocks to handle such situations, as shown below.
from ast import literal_eval
string_num = "7 + 8"
try:
integer_num = literal_eval(string_num)
except ValueError:
integer_num = 0
print(integer_num)
Here we assign 0 to integer_sum
if literal_eval()
throws a ValueError
exception.
The main advantage of literal_eval()
over the other two methods is that it is considered the safer option. Unlike eval()
, literal_eval()
does not execute arbitrary code or potentially harmful expressions. Furthermore, it handles errors better than int()
by raising more specific exceptions.
Discover how Caxita, an online travel engine application company with high workloads, eliminated downtime and allowed engineers to focus on development thanks to Cherry Servers' robust bare metal cloud and 24/7 technical support.
#How to convert int to string Python?
We can convert an integer value to a string value using the str()
function. This conversion method behaves similarly to int()
, except the result is a string value.
The str()
function automatically determines the base of the argument when a relevant prefix is specified, and it outputs the appropriate conversion to decimal format.
string = "100"
print(str(string))
print(type(str(string)))
binary = 0b100
print(str(binary))
print(type(str(binary)))
octal = 0o100
print(str(octal))
print(type(str(octal)))
hexadecimal = 0x100
print(str(hexadecimal))
print(type(str(hexadecimal)))
The main thing to be careful about here is that the str()
function does not throw exceptions. Therefore, you will need to handle any potential exceptions based on your specific use case.
#What should you be concerned about when converting strings to integers?
Overall, there are a few things to be cautious about when converting strings to integers, including:
- Verifying that the string contains a valid representation of an integer;
- Handling potential exceptions that may arise if the string includes non-numeric characters or is empty during the conversion process;
- Removing leading/trailing white spaces and any unwanted spaces using the
strip()
method; - Ensuring that your strings are in the correct numeric base, such as binary, octal, decimal, or hexadecimal;
- Dealing with positive or negative signs in the string representation;
- A string can contain characters that may not be in integers, which can cause exceptions. Use
try-except
blocks to catch any potential exceptions and handle them properly; - Although Python's
int
type doesn't have a practical size limit, handling large integers may consume significant resources and impact performance.
#Conclusion
Converting a string to an integer in Python is a task that every coder should be familiar with. In this article, we discuss three methods to accomplish this task, each with its own pros and cons.
We also explore the possible errors that may occur with each method and provide guidance on how to handle them. By practicing these methods for various use cases, you can encounter edge cases and develop problem-solving skills. Happy coding!
Cloud VPS Hosting
Starting at just $3.24 / month, get virtual servers with top-tier performance.